I just finished my first 2D game using AndEngine and want to share what I learned trough that journey.
So here are some tips for you. These tips are more about "Game Programing" in general, then about AndEngine, but I think you should know it before get started programming your first game.
Minimizing textures memory usage
There are many articles about textures, but here are some tips that I think you really should know.
Textures contains all your images of sprites, backgrounds, etc.. in your game.
Because images use large amount of memory you should know how to minimize that memory usage.
So what you can do to minimize memory usage?
Game resolution
Carefully choose resolution for your game. If you for example decide to have HD resolution (1920 x 1080) you will also need to create larger images, which will then occupy more memory. Also take into account that most mobile devices today (tables also), do not exceed resolution greater than 1280x720.
Lowering images quality
When it is possible save your images in lower quality (RGBA4444) instead of higher quality (RGBA8888).
Lower quality images can have problem with gradients and to resolve this you can enable dithering options in AndEngine.
To enable dithering on engine level, call setDithering(true) in onCreateEngineOptions()
public
EngineOptions onCreateEngineOptions()
{
engineOptions.getRenderOptions().setDithering(
true
);
}
Here you can see same image in RGBA8888 , RGBA4444 and RGBA4444 with dithering:
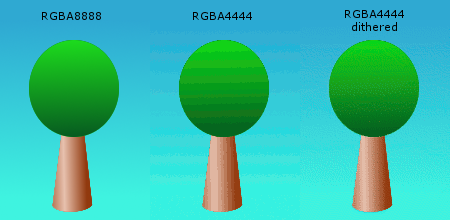
You can notice that gradient is not smooth on RGBA4444 without dithering.
Colorizing and scaling textures
There will be some cases in your game when you will need similar images which are different only by the scale or by the color. You can minimize your textures count by creating only one white/black texture which you can load into sprite and then change its scale or color.
Here you can see example of one white/black texture which is used for creating several different buttons with only setScale() and setColor() calls:
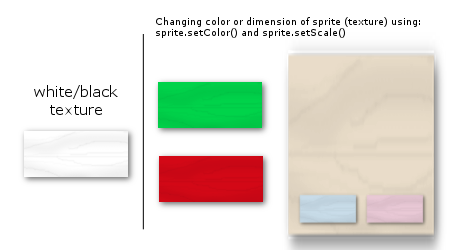
Composing sprites
When creating your backgrounds or sprites, its tempting to create whole image in your favorite image editor and load it as texture in AndEngine. This large image is usually created from many small similar images. Its better to try to create only small set of textures which you can use to compose larger images.
For example: game background can have many trees on it, but each tree is composed of trunks, branches and leaves. So instead creating several different trees and loading each of them as separate texture, you can only create image of trunk, several branches and leaves and load them as textures. Now composing these textures you can create several different type of trees. In composing your tree you can scale, rotate and colorize your textures.
Here is example of several different trees created by using only two textures.
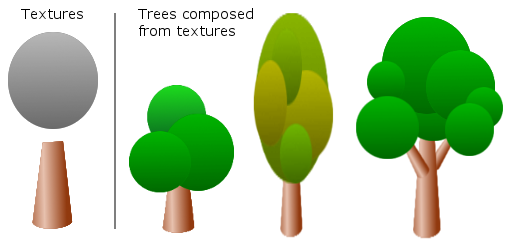
Texture size, Power of 2 rule
Keep in mind that when your texture will be load in graphic card memory, it will usually occupy "power of 2" size. For example if your texture size is 200x48 on the disk, in graphic card memory it will be kept as texture of size 256x64 (2^8 x 2^6). If its size is 260x260 on the disk, in graphic card memory it will bi kept as texture of size 512x512. This is significant loss of memory, so try to optimize your texure sizes having in mind "power of 2" rule.
Sprite sheets
Another options to avoid "power of 2" loss of memory is to create one large image, usually called "Sprite sheet", which will contain more textures combined together.
Here is example of one 256x256 sprite sheet:
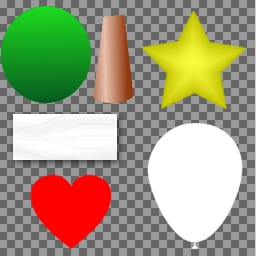
You can create sprite sheets manually, but I strongly not recommend it. There are some tools you can use for automating this process. One of such tools is TexturePacker which I used for my game.
Feel free to leave a comment or ask something if anything is not clear.
Also, if you have a time, you can download and review my game: Bubble Zoo Escape